graphx.h
#include <graphx.h>
The graphx
library implements efficient graphics routines from everything to drawing sprites, shapes, and tilemaps.
This allows a programmer to easily add quality graphics to their program without needing to worry about the low-level implementation details.
Overview
The graphx library places the LCD in a “palettized” mode - pixels on the screen are actually indexes into an array of colors rather than the color iself.
What this means is that graphx is only functionally capable of displaying up to 256 colors on-screen at one time.
This array of colors is called a “palette”, and is accessed using the gfx_palette
macro variable: e.g. the code gfx_palette[16]
accesses the 17th color in the palette, and can be directly read or written with a color value.
Palette colors are stored in 1555 format (a version of 565 format), and the macro gfx_RGBTo1555
may be used to convert a normal 24-bit RGB value into a palette color, for example gfx_palette[0] = gfx_RGBTo1555(10, 21, 32)
.
The function gfx_SetPalette
can set multiple color entries at once, which may be useful when configuring an entire custom color palette.
A better option when working with graphx is to utilize the convimg tool which is supplied with the toolchain. This tool is able to consume multiple images and sprites and to create a palette that matches as closely as possible with only 256 colors. The output of this tool is normal data arrays which are then automatically picked up by the compiler at build time, making the process seamless. Additionally, convimg allows sprite and color data to be stored in AppVars to help reduce the size of the program binary.
Default Palette
This is the default palette used by graphx, commonally known as the xlibc
palette for historical reasons.
This palette can be modified at any time with different colors, using either gfx_palette
or the output of the convimg tool.
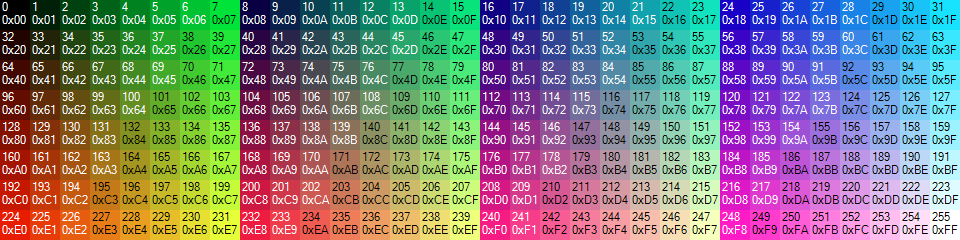
Coordinate System
The graphx library coordinate system consists of the horizontal (x
) and vertical (y
) components of the screen.
Pixel coordinates are generally expressed as an x
and y
pair, such as (x
, y
).
The graphx library uses the top left of the screen as pixel coordinate (0, 0), while the bottom right of the screen is pixel coordinate (319, 239).
The defines GFX_LCD_WIDTH
and GFX_LCD_HEIGHT
can be used to get the LCD screen width and height respectively.
Clipped vs. Unclipped
The graphx library contains many similar functions, e.g. gfx_Sprite
and gfx_Sprite_NoClip
.
As the name suggests, the _NoClip variant of a routine performs no clipping.
Clipping is the process of checking if parts (or all) of an object to be drawn are outside the window bounds and skipping drawing these out of bounds parts.
This checking process takes time.
If you can be sure that an object to be drawn is fully within the bounds of the screen, the clipping process can be safely skipped by using the _NoClip variant of the routine.
Do not use the _NoClip variant of a routine if you can not be sure that the object to be drawn is fully within the bounds of the screen. Doing so may result in corrupted graphics or memory. This is because the screen itself is represented as a region of memory. If you draw outside the bounds of that region, you risk overwriting other, possibly important data. This can cause corruption, and can cause crashes.
General Usage
To begin using the graphx library functions, the gfx_Begin
function must first be called.
This will configure the LCD in the proper mode, and set the color palette to the default palette described above.
Before the program exits, the function gfx_End
must be called in order to restore the LCD to the mode expected by the OS.
If this is not done, the screen may appear similar to the below image, which is what the OS looks like in palettized mode:
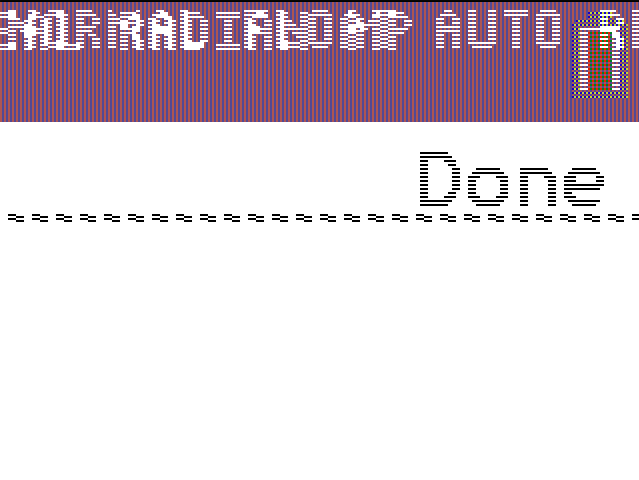
Partial Redraw
Partial redraw applies when you are moving a sprite against a static background. Sometimes, it can be too slow to redraw the entire screen just to move a small portion of it. Partial redraw saves a copy of what is behind the sprite, and draws it when the sprite moves again. For example, in a simple Pong game, if a white ball is drawn on a black background, instead of redrawing the entire screen each frame, you can redraw a small region around the ball to erase it.
Sample Implementation
A brief summation of the partial redraw using graphx is:
Allocate space for a temporary sprite.
When the background is drawn, a small copy of it is saved to the temporary sprite.
After the background is drawn, the sprite is drawn as normal.
Upon movement, the sprite is erased using the temporary sprite and the coordinates are updated.
The section of background with the updated coordinates is stored to the temporary sprite and the process repeated.
This is implemented using somewhat pseudo code:
int x = 0;
int y = 0;
gfx_sprite_t *behind_sprite = gfx_MallocSprite(SPRITE_WIDTH, SPRITE_HEIGHT);
// ...draw the background code here...
// set initial sprite state
gfx_GetSprite(behind_sprite, x, y);
gfx_TransparentSprite(sprite, x, y);
do {
// if we don't need to move, loop
if (no_move) continue;
// clear out the old sprite
gfx_Sprite(behind_sprite, x, y);
// ...movement code here...
// updates x and y
// get the new background and draw the moved sprite
gfx_GetSprite(behind_sprite, x, y);
gfx_TransparentSprite(sprite, x, y);
} while (moving);
Buffering Graphics
Buffering is a fancy method in order to prevent graphics from being displayed as they are being drawn. It is used primarily to eliminate visible draws which can make an application look amateurish, sluggish, or appear to flicker.
When graphics routines are buffered, they draw offscreen (non-visible) portion of memory, so the user doesn’t see the partial drawing. This is accomplished in one line with the following routine, usually placed directly after calling gfx_Begin():
gfx_SetDrawBuffer();
The next part is deciding how the drawn graphics should be displayed to the user.
Method 1 (Copying/Blitting)
The first method is to copy (commonly referred to as ‘blitting’) whatever is in the buffer to the visible screen. The simplest way is to call:
gfx_BlitBuffer();
Which will copy/blit the entire buffer to the screen. Alternatively, gfx_BlitLines() and gfx_BlitRectangle() and the other variations are used to specify the blitting bounds. This flickers less than normal drawing because having many small copy operations (like drawing sprites) is slower than one large copy operation (blitting the buffer to the screen). This means that a blit operation is less likely to get caught by a screen refresh than a bunch of sprite operations.
Method 2 (Buffer Swapping)
Buffer swapping swaps the visible screen with an offscreen buffer, leaving the contents on both. Whatever is currently on the screen will become the graphics buffer, and whatever is in the graphics buffer will be displayed on the screen. The code to swap the visible screen with non-visibile buffer is:
gfx_SwapDraw();
What actually happens is shown below, ‘graphics’ is simply where the graphics routines will draw to.
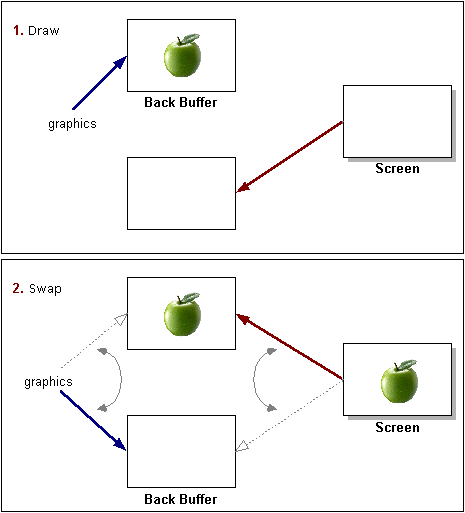
This method is really useful when you are redrawing all of the graphics each frame, and requires more work to handle if you only wish to do a partial redraw.
Pros and Cons
Buffering is slower than drawing to the main screen, but greatly improves perceived performance which is extremely important in graphical applications.
Blitting: Easy, simply a copy to the screen.
Swapping: Requires more programmer management (sometimes), faster than blitting.
Creating Sprites
Sprites are images that contain pixel data that can be drawn via graphx functions. The CE C Toolchain includes convimg, which is used to convert images in PNG or similar formats into binary data that can be used by graphx.
Getting Started
We are going to begin by taking a look at an example program to familiarize ourselves with how to run and configure convimg.
Open the graphx/sprites example, and navigate to the src/gfx folder.
The image oiram.png
is the sprite that wants to be converted, and convimg.yaml
is used to configure how the image should be converted.
Open convimg.yaml
in a text editor, which contains the below lines.
Run the command convimg --help
. This outputs the readme for convimg, and what each of the various commands do.
You can also find the readme online, available here.
palettes:
- name: global_palette
fixed-entries:
- color: {index: 0, r: 255, g: 0, b: 128}
- color: {index: 1, r: 255, g: 255, b: 255}
images: automatic
converts:
- name: sprites
palette: global_palette
transparent-color-index: 0
images:
- oiram.png
outputs:
- type: c
include-file: gfx.h
palettes:
- global_palette
converts:
- sprites
Converting Sprites
Converting sprites requires you to type make gfx
instead of make
.
Any time you change an image or the convimg.yaml
, you will need to execute this command.
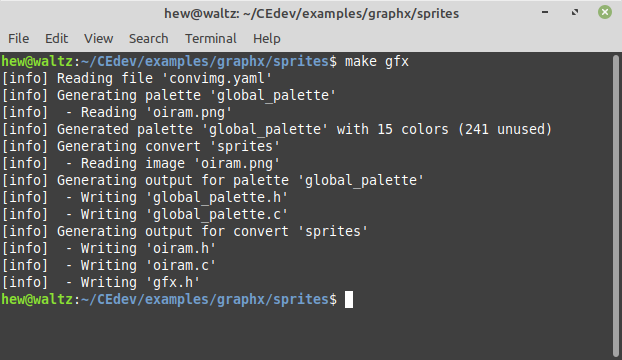
You can now make
the project as you would any of the other examples.
Explanation of output
Sprites used in the graphx library are allowed 8 bits per pixel (aka 8bpp), which allows for a total of 256
colors.
As shown in the previous image, convimg was able to create a suitable palette for oiram.png
with 15 colors.
Once the palette is generated, convimg outputs C headers and source files that are automatically picked up by the toolchain and compiled into the final program.
API Documentation
The below example template shows the best graphx buffer usage pattern:
// Standard #includes omitted
bool partial_redraw;
// Implement us!
void begin();
void end();
bool step();
void draw();
void main() {
begin(); // No rendering allowed!
gfx_Begin();
gfx_SetDrawBuffer(); // Draw to the buffer to avoid rendering artifacts
while (step()) { // No rendering allowed in step!
if (partial_redraw) // Only redraw part of the previous frame?
gfx_BlitScreen(); // Copy previous frame as a base for this frame
draw(); // As little non-rendering logic as possible
gfx_SwapDraw(); // Queue the buffered frame to be displayed
}
gfx_End();
end();
}
- Authors
Matt “MateoConLechuga” Waltz
Jacob “jacobly” Young
Zachary “Runer112” Wassall
Patrick “tr1p1ea” Prendergast
Peter “PT_” Tillema
”grosged”
Defines
-
gfx_palette
Direct LCD palette access via MMIO.
The palette has 256 entries, each 2 bytes in size.
-
gfx_vram
Direct LCD VRAM access.
Total of 153600 bytes in size.
-
GFX_LCD_WIDTH
Number of pixels wide the screen is.
-
GFX_LCD_HEIGHT
Number of pixels high the screen is.
-
gfx_vbuffer
Direct LCD VRAM access of the current buffer.
Total of 76800 bytes in size.
-
gfx_SetDrawBuffer()
Makes graphics routines act on the non-visible buffer.
-
gfx_SetDrawScreen()
Makes graphics routines act on the visible screen.
-
gfx_BlitScreen()
Copies the contents of the screen to the buffer.
-
gfx_BlitBuffer()
Copies the contents of the buffer to the screen.
-
gfx_MallocSprite(width, height)
Dynamically allocates memory for a sprite using
malloc
.width
andheight
will be set in the sprite. ReturnsNULL
upon allocation failure.See also
Note
If not used in a dynamic context and
width
andheight
are static, consider statically allocating the sprite instead with gfx_UninitedSprite() or gfx_TempSprite().- Parameters
width – [in] Sprite width.
height – [in] Sprite height.
- Returns
A pointer to the allocated sprite, or NULL if the allocation failed.
-
gfx_UninitedSprite(name, width, height)
Statically allocates uninitialized memory for a sprite.
Declares a
gfx_sprite_t *
with the givenname
pointing to the allocated memory.width
andheight
will not be set in the sprite, unlike gfx_TempSprite().See also
Warning
If used outside of a function body, the memory will be allocated in the global uninitialized data segment (BSS). If used inside a function body, the memory will be allocated on the stack. If the sprite is sufficiently large, usage inside a function body will overflow the stack, so it is recommended that this normally be used outside of a function body.
- Parameters
name – [in] Name of declared
gfx_sprite_t *
.width – [in] Sprite width.
height – [in] Sprite height.
-
gfx_TempSprite(name, width, height)
Statically allocates memory for a sprite.
Declares a
gfx_sprite_t *
with the givenname
pointing to the allocated memory.width
andheight
will be set in the sprite, unlike gfx_UninitedSprite().See also
- Attention
Due to
width
andheight
being set, the memory will be allocated in the initialized data segment. If the compiled program is not compressed, then this could be a serious source of bloat and gfx_UninitedSprite() should be preferred.
- Parameters
name – [in] Name of declared
gfx_sprite_t *
.width – [in] Sprite width.
height – [in] Sprite height.
-
gfx_AllocRLETSprite(data_size, malloc_routine)
Dynamically allocates memory for a sprite with RLE transparency.
Allocates the memory with
malloc_routine
. ReturnsNULL
upon allocation failure.data_size
is the maximum predicted/calculated size of the sprite data (excluding the width and height bytes) that will be stored in the allocated sprite. Sprite data size could be up to(width + 1) * height * 3 / 2
bytes in the worst case, in which pixels horizontally alternate between non-transparent and transparent and each row begins with a non-transparent pixel. But if the average length of a horizontal transparent run is at least 2, then the sprite data will be no larger than(width + 1) * height
bytes. The exact data size necessary is2 * hr + nont + rnont - ret
bytes, where:hr
: Number of horizontal transparent runs.nont
: Number of non-transparent runs.rnont
: Number of rows beginning with a non-transparent pixel.rbnont
: Number of rows beginning with a non-transparent pixel.ret
: Number of rows ending with a transparent pixel.Remark
If using
malloc
as themalloc_routine
, gfx_MallocRLETSprite() can be used as a shortcut.Note
If not used in a dynamic context and
data_size
is static, consider statically allocating the sprite instead with gfx_UninitedRLETSprite() or gfx_TempRLETSprite().- Parameters
data_size – [in] (Maximum) sprite data size.
malloc_routine – [in] Malloc implementation to use.
- Returns
A pointer to the allocated sprite, or NULL if the allocation failed..
-
gfx_MallocRLETSprite(data_size)
Dynamically allocates memory for a sprite with RLE transparency using
malloc
.data_size
is the size to allocate for sprite data; see gfx_AllocRLETSprite() for information. ReturnsNULL
upon allocation failure.See also
Note
If not used in a dynamic context and
data_size
is static, consider statically allocating the sprite instead with gfx_UninitedRLETSprite() or gfx_TempRLETSprite().- Parameters
data_size – [in] (Maximum) sprite data size.
- Returns
A pointer to the allocated sprite, or NULL if the allocation failed.
-
gfx_UninitedRLETSprite(name, data_size)
Statically allocates uninitialized memory for a sprite with RLE transparency.
Declares a
gfx_rletsprite_t *
with the givenname
pointing to the allocated memory.data_size
is the size to allocate for sprite data; see gfx_AllocRLETSprite() for information.See also
Warning
If used outside of a function body, the memory will be allocated in the global uninitialized data segment (BSS). If used inside a function body, the memory will be allocated on the stack. If the sprite is sufficiently large, usage inside a function body will overflow the stack, so it is recommended that this normally be used outside of a function body.
- Parameters
name – [in] Name of declared
gfx_rletsprite_t *
.data_size – [in] (Maximum) sprite data size.
-
gfx_SetTile(tilemap, x_offset, y_offset, value)
Sets a particular tile’s sprite tileset index.
This function bases position on the pixel offset from the top-left of the tilemap.
- Parameters
tilemap – Pointer to initialized tilemap structure.
x_offset – Offset in pixels from the left of the tilemap.
y_offset – Offset in pixels from the top of the tilemap.
value – Sprite index in tileset.
-
gfx_GetTile(tilemap, x_offset, y_offset)
Gets a particular tile’s sprite tileset index.
This function bases position on the pixel offset from the top-left of the tilemap.
- Parameters
tilemap – [in] Pointer to an initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
gfx_SetTileMapped(tilemap, col, row, value)
Sets a particular tile’s sprite tileset index.
This function uses the corrdinates from the tilemap array.
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
col – [in] Column of tile in tilemap.
row – [in] Row of tile in tilemap.
value – [in] Sprite index in tileset.
-
gfx_GetTileMapped(tilemap, col, row)
Gets a particular tile’s sprite tileset index.
This function uses the corrdinates from the tilemap array.
- Parameters
tilemap – [in] Pointer to an initialized tilemap structure.
col – [in] Column of tile in tilemap.
row – [in] Row of tile in tilemap.
-
gfx_Circle_NoClip(x, y, radius)
Draws an unclipped circle outline.
Performs faster than using gfx_Circle, but can cause corruption if used outside the bounds of the screen.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
radius – [in] The radius of the circle.
-
gfx_GetSprite_NoClip(sprite_buffer, x, y)
Grabs the background behind an unclipped sprite.
This is useful for partial redraw.
Note
sprite_buffer
must be pointing to a large enough buffer to hold (width * height + 2) number of bytes.- Parameters
sprite_buffer – [out] Buffer used to store grabbed sprite.
x – [in] X coordinate to grab sprite.
y – [in] Y coordinate to grab sprite.
- Returns
A pointer to sprite_buffer.
-
gfx_RotatedTransparentSprite_NoClip(sprite, x, y, angle)
Helper macro to only perform rotation using gfx_RotatedScaledTransparentSprite_NoClip.
- Parameters
sprite – [in] Input sprite to rotate/scale.
x – [in] X coordinate position.
y – [in] Y coordinate position.
angle – [in] 256 position angular integer.
-
gfx_RotatedSprite_NoClip(sprite, x, y, angle)
Helper macro to only perform rotation using gfx_RotatedScaledSprite_NoClip.
- Parameters
sprite – [in] Input sprite to rotate/scale.
x – [in] X coordinate position.
y – [in] Y coordinate position.
angle – [in] 256 position angular integer.
-
gfx_RotateSprite(sprite_in, sprite_out, angle)
Helper macro to only perform rotation using gfx_RotateScaleSprite.
See also
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to rotate.
sprite_out – [out] Pointer to where rotated sprite will be stored.
angle – [in] 256 position angular integer.
- Returns
A pointer to
sprite_out
.
-
gfx_ConvertMallocRLETSprite(sprite_in)
Converts a sprite with normal transparency to a sprite with RLE transparency, allocating the exact amount of necessary space for the converted sprite using
malloc
.Width and height will be set in the converted sprite. Returns
NULL
upon allocation failure.The transparent color index in the input sprite is controlled by gfx_SetTransparentColor().
Remark
A gfx_sprite_t can be converted into an appropriately large, already-allocated gfx_rletsprite_t using gfx_ConvertToRLETSprite().
See also
- Parameters
sprite_in – [in] Input sprite with normal transparency.
- Returns
A newly allocated converted sprite with RLE transparency.
-
gfx_RGBTo1555(r, g, b)
Converts an RGB value to a palette color.
Note
Conversion is not 100% perfect, but is good enough in most cases.
- Parameters
r – [in] Red value (0-255).
g – [in] Green value (0-255).
b – [in] Blue value (0-255).
-
gfx_CheckRectangleHotspot(x0, y0, w0, h0, x1, y1, w1, h1)
Tests if a rectangular area intersects/collides with another rectangular area.
- Parameters
x0 – [in] Rectangle 0 X coordinate
y0 – [in] Rectangle 0 Y coordinate
w0 – [in] Rectangle 0 width
h0 – [in] Rectangle 0 height
x1 – [in] Rectangle 1 X coordinate
y1 – [in] Rectangle 1 Y coordinate
w1 – [in] Rectangle 1 width
h1 – [in] Rectangle 1 height
- Returns
true if the rectangles are intersecting.
-
gfx_GetZX7SpriteWidth(zx7_sprite)
-
gfx_GetZX7SpriteHeight(zx7_sprite)
-
gfx_GetZX7SpriteSize(zx7_sprite)
Calculates the amount of memory that a zx7-compressed sprite would use when decompressed.
Sprite size is calculated as 2 + (width * height).
ZX7 data always starts with a literal, which is the sprite’s width. The next byte contains flags, which indicates if the following bytes are literals or codewords. If bit 7 of that is zero, the byte immediately after it is a literal and can be read in as sprite height. Otherwise, the bits that follows indicates a codeword, making sprite height the same as width.
- Parameters
zx7_sprite – [in] ZX7-compressed sprite
- Returns
Size, in bytes, of decompressed sprite
Typedefs
-
typedef struct gfx_sprite_t gfx_sprite_t
Sprite (image) type.
Whether or not a sprite includes transparency is not explicitly encoded, and is determined only by usage. If used with transparency, transparent pixels are those with a certain color index, which can be set with gfx_SetTransparentColor().
Remark
Create at compile-time with a tool like convimg. Create at runtime (with uninitialized data) with gfx_MallocSprite(), gfx_UninitedSprite(), or gfx_TempSprite().
Note
Displaying a gfx_rletsprite_t (which includes transparency) is significantly faster than displaying a gfx_sprite_t with transparency, and should be preferred. However, gfx_rletsprite_t does not support transformations, such as flipping and rotation. Such transformations can be applied to a gfx_sprite_t, which can then be converted to a gfx_rletsprite_t for faster display using gfx_ConvertToNewRLETSprite() or gfx_ConvertToRLETSprite().
-
typedef struct gfx_rletsprite_t gfx_rletsprite_t
Sprite (image) type with RLE transparency.
Remark
Create at compile-time with a tool like convimg.
- Attention
Displaying a gfx_rletsprite_t (which includes transparency) is significantly faster than displaying a gfx_sprite_t with transparency, and should be preferred. However, gfx_rletsprite_t does not support transformations, such as flipping and rotation. Such transformations can be applied to a gfx_sprite_t, which can then be converted to a gfx_rletsprite_t for faster display using gfx_ConvertToNewRLETSprite() or gfx_ConvertToRLETSprite().
-
typedef struct gfx_point_t gfx_point_t
A structure for working with 2D points.
-
typedef struct gfx_region_t gfx_region_t
Defines a rectangular graphics region.
See also
-
typedef struct gfx_tilemap_t gfx_tilemap_t
Defines tilemap structure.
See also
Enums
-
enum gfx_mode_t
Stores operating modes of this library.
See also
Values:
-
enumerator gfx_8bpp = 0x27
Enable 8 bits per pixel mode.
-
enumerator gfx_8bpp = 0x27
-
enum gfx_tilemap_type_t
Used for defining tile types.
See also
Values:
-
enumerator gfx_tile_no_pow2 = 0
Use when the tile width/height is not a power of 2.
-
enumerator gfx_tile_2_pixel
Use when the tile width/height is 2 pixels.
-
enumerator gfx_tile_4_pixel
Use when the tile width/height is 4 pixels.
-
enumerator gfx_tile_8_pixel
Use when the tile width/height is 8 pixels.
-
enumerator gfx_tile_16_pixel
Use when the tile width/height is 16 pixels.
-
enumerator gfx_tile_32_pixel
Use when the tile width/height is 32 pixels.
-
enumerator gfx_tile_64_pixel
Use when the tile width/height is 64 pixels.
-
enumerator gfx_tile_128_pixel
Use when the tile width/height is 128 pixels.
-
enumerator gfx_tile_no_pow2 = 0
Functions
-
void gfx_Begin()
Initializes the
graphx
library context.This function should be called before any other
graphx
library routines.
-
void gfx_End(void)
Ends the
graphx
library context.Restores the LCD to 16bpp and clears the screen. 16bpp is used by the OS, so if you don’t call this, the screen will look weird and won’t work right.
-
gfx_sprite_t *gfx_AllocSprite(uint8_t width, uint8_t height, void *(*malloc_routine)(size_t)) __attribute__((__nonnull__(3)))
Dynamically allocates memory for a sprite.
Allocates the memory with
malloc_routine
.width
andheight
will be set in the allocated sprite. ReturnsNULL
upon allocation failure.Remark
If using
malloc
as themalloc_routine
, gfx_MallocSprite() can be used as a shortcut.Note
If not used in a dynamic context and
width
andheight
are static, consider statically allocating the sprite instead with gfx_UninitedSprite() or gfx_TempSprite().- Parameters
width – [in] Sprite width.
height – [in] Sprite height.
malloc_routine – [in] Malloc implementation to use.
- Returns
A pointer to the allocated sprite.
-
void gfx_Tilemap(const gfx_tilemap_t *tilemap, uint24_t x_offset, uint24_t y_offset)
Draws a tilemap.
See also
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
void gfx_Tilemap_NoClip(const gfx_tilemap_t *tilemap, uint24_t x_offset, uint24_t y_offset)
Draws an unclipped tilemap.
See also
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
void gfx_TransparentTilemap(const gfx_tilemap_t *tilemap, uint24_t x_offset, uint24_t y_offset)
Draws a transparent tilemap.
See also
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
void gfx_TransparentTilemap_NoClip(const gfx_tilemap_t *tilemap, uint24_t x_offset, uint24_t y_offset)
Draws an unclipped transparent tilemap.
See also
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
uint8_t *gfx_TilePtr(const gfx_tilemap_t *tilemap, uint24_t x_offset, uint24_t y_offset) __attribute__((__pure__))
Gets a pointer to a particular sprite tileset index.
This function bases position on the pixel offset from the top-left of the tilemap.
- Parameters
tilemap – [in] Pointer to initialized tilemap structure.
x_offset – [in] Offset in pixels from the left of the tilemap.
y_offset – [in] Offset in pixels from the top of the tilemap.
-
uint8_t *gfx_TilePtrMapped(const gfx_tilemap_t *tilemap, uint8_t col, uint8_t row) __attribute__((__pure__))
Gets a pointer to a particular sprite tileset index.
This function uses the corrdinates from the tilemap array.
- Parameters
tilemap – [in] Pointer to an initialized tilemap structure.
col – [in] Column of tile in tilemap.
row – [in] Row of tile in tilemap.
-
uint8_t gfx_SetColor(uint8_t index)
Sets the color index that drawing routines will use.
This applies to lines, rectangles, circles, etc.
- Parameters
index – [in] Color index to set.
- Returns
Previous set color index.
-
uint8_t gfx_SetTransparentColor(uint8_t index)
Sets the transparent index that drawing routines will use.
This currently applies to transparent sprites, both scaled and unscaled
- Parameters
index – [in] Transparent color index to set.
- Returns
The previous transparent color index.
-
void gfx_SetDefaultPalette(gfx_mode_t mode)
Sets up the default palette for the given mode.
- Parameters
mode – [in] Palette to use.
-
void gfx_SetPalette(const void *palette, uint24_t size, uint8_t offset)
Sets entries in the palette.
- Parameters
palette – [in] Pointer to palette to set.
size – [in] Size of palette in bytes.
offset – [in] Palette index to insert at.
-
void gfx_FillScreen(uint8_t index)
Fills the screen with a given palette index.
- Parameters
index – [in] Pallete index to fill with.
-
void gfx_ZeroScreen(void)
Implements a faster version of gfx_FillScreen(0).
See also
-
void gfx_SetPixel(uint24_t x, uint8_t y)
Sets a pixel to the global color index.
See also
Note
Pixels are only clipped within the screen boundaries, not the clip region.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
-
uint8_t gfx_GetPixel(uint24_t x, uint8_t y)
Gets a pixel’s color index.
Note
Pixels are only clipped within the screen boundaries, not the clip region.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_Line(int x0, int y0, int x1, int y1)
Draws a line.
- Parameters
x0 – [in] First X coordinate.
y0 – [in] First Y coordinate.
x1 – [in] Second X coordinate.
y1 – [in] Second Y coordinate.
-
void gfx_Line_NoClip(uint24_t x0, uint8_t y0, uint24_t x1, uint8_t y1)
Draws an unclipped line.
- Parameters
x0 – [in] First X coordinate.
y0 – [in] First Y coordinate.
x1 – [in] Second X coordinate.
y1 – [in] Second Y coordinate.
-
void gfx_HorizLine(int x, int y, int length)
Draws a horizontal line.
This routine is faster than gfx_Line for horizontal lines.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
length – [in] Length of line.
-
void gfx_HorizLine_NoClip(uint24_t x, uint8_t y, uint24_t length)
Draws an unclipped horizontal line.
This routine is faster than gfx_Line_NoClip for horizontal lines.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
length – [in] Length of line.
-
void gfx_VertLine(int x, int y, int length)
Draws a vertical line.
This routine is faster than gfx_Line for vertical lines.
- Parameters
x – [in] X coordinate
y – [in] Y coordinate
length – [in] Length of line
-
void gfx_VertLine_NoClip(uint24_t x, uint8_t y, uint24_t length)
Draws an unclipped vertical line.
This routine is faster than gfx_Line_NoClip for vertical lines.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
length – [in] Length of line.
-
void gfx_Rectangle(int x, int y, int width, int height)
Draws a rectangle outline.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
width – [in] Width of rectangle.
height – [in] Height of rectangle.
-
void gfx_Rectangle_NoClip(uint24_t x, uint8_t y, uint24_t width, uint8_t height)
Draws an unclipped rectangle outline.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
width – [in] Width of rectangle.
height – [in] Height of rectangle.
-
void gfx_FillRectangle(int x, int y, int width, int height)
Draws a filled rectangle.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
width – [in] Width of rectangle.
height – [in] Height of rectangle.
-
void gfx_FillRectangle_NoClip(uint24_t x, uint8_t y, uint24_t width, uint8_t height)
Draws an unclipped filled rectangle.
- Parameters
x – [in] X coordinate
y – [in] Y coordinate
width – [in] Width of rectangle
height – [in] Height of rectangle
-
void gfx_Circle(int x, int y, uint24_t radius)
Draws a circle outline.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
radius – [in] The radius of the circle.
-
void gfx_FillCircle(int x, int y, uint24_t radius)
Draws a filled circle.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
radius – [in] The radius of the circle.
-
void gfx_FillCircle_NoClip(uint24_t x, uint8_t y, uint24_t radius)
Draws an unclipped filled circle.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
radius – [in] The radius of the circle.
-
void gfx_FillEllipse_NoClip(uint24_t x, uint24_t y, uint8_t a, uint8_t b)
Draws an unclipped filled ellipse.
- Parameters
x – [in] X coordinate of the center.
y – [in] Y coordinate of the center.
a – [in] The horizontal radius of the ellipse (current maximum is 128).
b – [in] The vertical radius of the ellipse (current maximum is 128).
-
void gfx_FillEllipse(int24_t x, int24_t y, uint24_t a, uint24_t b)
Draws a filled ellipse.
- Parameters
x – [in] X coordinate of the center.
y – [in] Y coordinate of the center.
a – [in] The horizontal radius of the ellipse (current maximum is 128).
b – [in] The vertical radius of the ellipse (current maximum is 128).
-
void gfx_Ellipse_NoClip(uint24_t x, uint24_t y, uint8_t a, uint8_t b)
Draws an unclipped ellipse.
- Parameters
x – [in] X coordinate of the center.
y – [in] Y coordinate of the center.
a – [in] The horizontal radius of the ellipse (current maximum is 128).
b – [in] The vertical radius of the ellipse (current maximum is 128).
-
void gfx_Ellipse(int24_t x, int24_t y, uint24_t a, uint24_t b)
Draws an ellipse.
- Parameters
x – [in] X coordinate of the center.
y – [in] Y coordinate of the center.
a – [in] The horizontal radius of the ellipse (current maximum is 128).
b – [in] The vertical radius of the ellipse (current maximum is 128).
-
void gfx_Polygon(const int *points, unsigned num_points)
Draws a clipped polygon outline.
int points[6] = { 160, 1, // (x0, y0) 1, 238, // (x1, y1) 318,238, // (x2, y2) }; num_points = 3; gfx_Polygon(points, num_points);
- Parameters
points – [in] Pointer to x and y pairs.
num_points – [in] Number of x and y pairs.
-
void gfx_Polygon_NoClip(const int *points, unsigned num_points)
Draws an unclipped polygon outline.
int points[6] = { 160, 1, // (x0, y0) 1, 238, // (x1, y1) 318,238, // (x2, y2) }; num_points = 3; gfx_Polygon_NoClip(points, num_points)
- Parameters
points – [in] Pointer to x and y pairs
num_points – [in] Number of x and y pairs
-
void gfx_FillTriangle(int x0, int y0, int x1, int y1, int x2, int y2)
Draws a clipped filled triangle.
- Parameters
x0 – [in] First X coordinate.
y0 – [in] First Y coordinate.
x1 – [in] Second X coordinate.
y1 – [in] Second Y coordinate.
x2 – [in] Third X coordinate.
y2 – [in] Third Y coordinate.
-
void gfx_FillTriangle_NoClip(int x0, int y0, int x1, int y1, int x2, int y2)
Draws a unclipped filled triangle.
- Parameters
x0 – [in] First X coordinate.
y0 – [in] First Y coordinate.
x1 – [in] Second X coordinate.
y1 – [in] Second Y coordinate.
x2 – [in] Third X coordinate.
y2 – [in] Third Y coordinate.
-
void gfx_SetDraw(uint8_t location)
Forces all graphics routines draw location.
See also
- Parameters
location – [in] Location routines should draw to.
-
uint8_t gfx_GetDraw(void)
Gets the current drawing buffer.
See also
- Returns
Location type enumeration.
-
void gfx_SwapDraw(void)
Swaps the roles of the screen and drawing buffers.
Does not wait for the old screen buffer to finish being displayed. Instead, the next invocation of a graphx drawing function will block, (pause program execution) waiting for this event. To block and wait explicitly, use gfx_Wait().
The LCD driver maintains its own screen buffer pointer for the duration of a refresh. The swap performed by this function will only be picked up at a point between refreshes.
Remark
In practice, this function should be invoked immediately after finishing drawing a frame to the drawing buffer, and invocation of the first graphx drawing function for the next frame should be scheduled as late as possible relative to non-drawing logic. Non-drawing logic can execute during time when a drawing function may otherwise block.
-
void gfx_Wait(void)
Waits for the screen buffer to finish being displayed after gfx_SwapDraw().
Remark
In practice, this function should not need to be invoked by user code. It should be invoked by custom drawing functions (as late as reasonably possible) before writing to the drawing buffer, gfx_vbuffer.
-
void gfx_Blit(gfx_location_t src)
Copies the input buffer to the opposite buffer.
No clipping is performed as it is a copy not a draw.
- Parameters
src – [in] drawing location to copy from.
-
void gfx_BlitLines(gfx_location_t src, uint8_t y_loc, uint8_t num_lines)
Copies lines from the input buffer to the opposite buffer.
No clipping is performed as it is a copy not a draw.
See also
- Parameters
src – [in] drawing location to copy from.
y_loc – [in] Y Location to begin copying at.
num_lines – [in] Number of lines to copy.
-
void gfx_BlitRectangle(gfx_location_t src, uint24_t x, uint8_t y, uint24_t width, uint24_t height)
Transfers a rectangle from the source graphics buffer to the opposite buffer.
No clipping is performed as it is a copy not a draw.
See also
- Parameters
src – [in] drawing location to copy from.
x – [in] X coordinate.
y – [in] Y coordinate.
width – [in] Width of rectangle.
height – [in] Height of rectangle.
-
void gfx_CopyRectangle(gfx_location_t src, gfx_location_t dst, uint24_t src_x, uint8_t src_y, uint24_t dst_x, uint8_t dst_y, uint24_t width, uint8_t height)
Copies a rectangular region between graphics buffers or to the same graphics buffer.
The behavior is undefined when the rectangles overlap. No clipping is performed as it is a copy not a draw.
See also
- Parameters
src – [in] Graphics buffer to copy from.i
dst – [in] Graphics buffer to copy to.
src_x – [in] X coordinate on src.
src_y – [in] Y coordinate on src.
dst_x – [in] X coordinate on dst.
dst_y – [in] Y coordinate on dst.
width – [in] Width of rectangle.
height – [in] Height of rectangle.
-
void gfx_SetTextScale(uint8_t width_scale, uint8_t height_scale)
Sets the scaling for text.
Scaling is performed by multiplying the width / height of the currently loaded text by the supplied scaling factors.
Values in the range from 1-5 are preferred.
- Parameters
width_scale – [in] Text width scaling
height_scale – [in] Text height scaling
-
void gfx_PrintChar(const char c)
Prints a single character.
Outputs a character at the current cursor position.
Note
By default, no text clipping is performed. See gfx_SetTextConfig.
- Parameters
c – [in] Character to print
-
void gfx_PrintInt(int n, uint8_t length)
Prints a signed integer.
Outputs at the current cursor position. Pads the integer with leading zeros if necessary to satisfy the specified minimum length. For example, gfx_PrintInt(5,3) prints “005”.
Note
By default, no text clipping is performed. See gfx_SetTextConfig.
Note
length
must be between 1 and 8, inclusive- Parameters
n – [in] Integer to print.
length – [in] Minimum number of characters to print.
-
void gfx_PrintUInt(unsigned int n, uint8_t length)
Prints an unsigned integer.
Outputs at the current cursor position. Pads the integer with leading zeros if necessary to satisfy the specified minimum length. For example, gfx_PrintUInt(5,3) prints “005”.
Note
By default, no text clipping is performed. See gfx_SetTextConfig.
Note
length
must be between 1 and 8, inclusive- Parameters
n – [in] Unsigned integer to print.
length – [in] Minimum number of characters to print.
-
void gfx_PrintString(const char *string)
Prints a string.
Outputs a string at the current cursor position.
Note
By default, no text clipping is performed. See gfx_SetTextConfig.
- Parameters
string – [in] Pointer to string to print.
-
void gfx_PrintStringXY(const char *string, int x, int y)
Prints a string at a specific location.
Outputs a string at the supplied coordinates. This has the same effect as calling gfx_SetTextXY(x,y); then gfx_PrintString();
Note
By default, no text clipping is performed. See gfx_SetTextConfig.
- Parameters
string – [in] Pointer to string to print.
x – [in] X coordinate.
y – [in] Y coordinate.
-
int gfx_GetTextX(void) __attribute__((__pure__))
- Returns
The current text cursor X position.
-
int gfx_GetTextY(void) __attribute__((__pure__))
- Returns
The current text cursor Y position.
-
void gfx_SetTextXY(int x, int y)
Sets the text cursor X and Y positions.
- Parameters
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_SetTextConfig(uint8_t config)
Sets the configuration for the text routines.
See also
Note
Scaled text does not clip
- Parameters
config – [in] Set the options for the text mode.
-
uint8_t gfx_SetTextFGColor(uint8_t color)
Sets the text foreground color.
Note
Default text foreground palette index: 0.
Note
Default text background palette index is 255, so if you don’t change it and try to draw with color 255, nothing will happen. You can change this with gfx_SetTextTransparentColor(color).
- Parameters
color – [in] Palette index to set.
- Returns
Previous text foreground palette index.
-
uint8_t gfx_SetTextBGColor(uint8_t color)
Sets the text background (highlight) color.
Note
Default text background palette index: 255. (default transparent color)
- Parameters
color – [in] Palette index to set.
- Returns
Previous text background palette index.
-
uint8_t gfx_SetTextTransparentColor(uint8_t color)
Sets the text transparency color.
Note
Default text transparency palette index: 255.
- Parameters
color – [in] Palette index to set.
- Returns
Previous text transparency palette index.
-
void gfx_Sprite(const gfx_sprite_t *sprite, int x, int y)
Draws a sprite.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_Sprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y)
Draws an unclipped sprite.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_TransparentSprite(const gfx_sprite_t *sprite, int x, int y)
Draws a transparent sprite.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_TransparentSprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y)
Draws an unclipped transparent sprite.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
-
gfx_sprite_t *gfx_GetSprite(gfx_sprite_t *sprite_buffer, int x, int y)
Grabs the background behind a sprite.
This is useful for partial redraw.
Note
sprite_buffer
must be pointing to a large enough buffer to hold (width * height + 2) number of bytes.- Parameters
sprite_buffer – [out] Buffer used to store grabbed sprite.
x – [in] X coordinate to grab sprite.
y – [in] Y coordinate to grab sprite.
- Returns
A pointer to sprite_buffer.
-
void gfx_ScaledSprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y, uint8_t width_scale, uint8_t height_scale)
Scales an unclipped sprite.
Scaling factors must be greater than or equal to 1, and an integer factor. Sprites are scaled by multiplying the dimensions by the respective factors.
Note
Usable with gfx_GetSprite in order to create clipped versions.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
width_scale – [in] Width scaling factor.
height_scale – [in] Height scaling factor.
-
void gfx_ScaledTransparentSprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y, uint8_t width_scale, uint8_t height_scale)
Scales an unclipped transparent sprite.
Scaling factors must be greater than or equal to 1, and an integer factor. Sprites are scaled by multiplying the dimensions by the respective factors.
Note
Usable with gfx_GetSprite in order to create clipped versions.
- Parameters
sprite – [in] Pointer to an initialized sprite structure.
x – [in] X coordinate.
y – [in] Y coordinate.
width_scale – [in] Width scaling factor.
height_scale – [in] Height scaling factor.
-
uint8_t gfx_RotatedScaledTransparentSprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y, uint8_t angle, uint8_t scale)
Fixed Rotation with scaling factor for sprites.
Note
A scale factor of 64 represents 100% scaling.
Note
Sprites larger than 210x210 may have rendering artifacts.
Warning
This routine only accepts square input sprites.
Warning
The output size cannot be greater than 255x255.
- Parameters
sprite – [in] Input sprite to rotate/scale.
x – [in] X coordinate position.
y – [in] Y coordinate position.
angle – [in] 256 position angular integer.
scale – [in] Scaling factor; range is about 1% to 400% scale.
- Returns
The size of the sprite after scaling. This can be used for centering purposes.
-
uint8_t gfx_RotatedScaledSprite_NoClip(const gfx_sprite_t *sprite, uint24_t x, uint8_t y, uint8_t angle, uint8_t scale)
Fixed Rotation with scaling fator for sprites without transparency.
Note
A scale factor of 64 represents 100% scaling.
Note
Sprites larger than 210x210 may have rendering artifacts.
Warning
This routine only accepts square input sprites.
Warning
The output size cannot be greater than 255x255.
- Parameters
sprite – [in] Input sprite to rotate/scale.
x – [in] X coordinate position.
y – [in] Y coordinate position.
angle – [in] 256 position angular integer.
scale – [in] Scaling factor; range is about 1% to 400% scale. 64 represents 100% scaling.
- Returns
The size of the sprite after scaling. This can be used for centering purposes.
- gfx_sprite_t * gfx_FlipSpriteX (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Flips a sprite along the X axis.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to flip.
sprite_out – [out] Pointer to where flipped sprite will be stored.
- Returns
A pointer to sprite_out.
- gfx_sprite_t * gfx_FlipSpriteY (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Flips a sprite along the Y axis.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to flip.
sprite_out – [out] Pointer to where flipped sprite will be stored.
- Returns
A pointer to sprite_out.
- gfx_sprite_t * gfx_RotateSpriteC (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Rotates a sprite 90 degrees clockwise.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to rotate.
sprite_out – [out] Pointer to where rotated sprite will be stored.
- Returns
A pointer to sprite_out.
- gfx_sprite_t * gfx_RotateSpriteCC (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Rotates a sprite 90 degrees counter clockwise.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to rotate.
sprite_out – [out] Pointer to where rotated sprite will be stored.
- Returns
A pointer to sprite_out.
- gfx_sprite_t * gfx_RotateSpriteHalf (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Rotates a sprite 180 degrees.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to rotate.
sprite_out – [out] Pointer to where rotated sprite will be stored.
- Returns
A pointer to sprite_out.
- gfx_sprite_t * gfx_ScaleSprite (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out)
Resizes a sprite to new dimensions.
Place new image dimensions in sprite_out; i.e. sprite_out->width = 80; sprite_out->height = 20.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
- Parameters
sprite_in – [in] Input sprite to scale.
sprite_out – [out] Pointer to where scaled sprite will be stored.
- Returns
A pointer to
sprite_out
.
- gfx_sprite_t * gfx_RotateScaleSprite (const gfx_sprite_t *__restrict sprite_in, gfx_sprite_t *__restrict sprite_out, uint8_t angle, uint8_t scale)
Fixed Rotation with scaling factor for sprites.
The output sprite is updated with the dimensions required for the implemented scaling factor. You must make sure that
sprite_out
has enough memory to store the needed output sprite. This can be found with the following formula: size = (max_scale / 64) * width * height + 2;Note
A scale factor of 64 represents 100% scaling.
Note
Rendering artifacts may occur if the output is larger than 210x210.
Note
sprite_in and sprite_out cannot be the same. Ensure sprite_out is allocated.
Warning
This routine only accepts square input sprites.
Warning
The output size cannot be greater than 255x255.
- Parameters
sprite_in – [in] Input sprite to rotate/scale.
sprite_out – [out] Pointer to where rotated/scaled sprite will be stored.
angle – [in] 256 position angular integer.
scale – [in] Scaling factor; range is about 1% to 400% scale.
- Returns
A pointer to
sprite_out
.
-
gfx_sprite_t *gfx_GetSpriteChar(char c)
Creates a temporary character sprite.
This may be useful for performing rotations and other. operations on characters. The sprite returned is always 8x8 pixels.
- Parameters
c – [in] Character to generate.
- Returns
A sprite of the character data.
-
uint8_t *gfx_SetFontData(const uint8_t *data)
Sets the font’s character data.
Fonts can be created manually or and exported to a C-style format using 8x8 Pixel ROM Font Editor: (https://www.min.at/prinz/o/software/pixelfont/#download)
Note
Format of font data is 8 bytes horizontally aligned.
- Parameters
data – [in] Pointer to formatted 8x8 pixel font.
- Returns
Pointer to previous font data.
-
uint8_t *gfx_SetCharData(uint8_t index, const uint8_t *data)
Sets the font data for a specific character.
See also
Note
Format of font data is 8 bytes horizontally aligned.
- Parameters
index – [in] Character index to modify. (if using default font, values range from 0-127, custom font can have indexes 0-255).
data – [in] Pointer to formatted 8x8 pixel font.
- Returns
Pointer to current character data if
data
is NULL, otherwise a pointer to next character data.
-
void gfx_SetFontSpacing(const uint8_t *spacing)
Sets the font spacing for each character.
Format is an array of bytes, where each index in the array corresponds to the character’s numerical value.
- Parameters
spacing – [in] Pointer to array of character spacing.
-
uint8_t gfx_SetFontHeight(uint8_t height)
Sets the height in pixels of each character.
The default value is 8 pixels.
- Parameters
height – [in] New font height in pixels.
- Returns
Previous height of font in pixels.
-
void gfx_SetMonospaceFont(uint8_t spacing)
Sets a monospaced font width.
Note
To disable monospaced font, set to 0.
- Parameters
spacing – [in] Distance between characters.
-
unsigned int gfx_GetStringWidth(const char *string) __attribute__((__pure__))
Gets the pixel width of the given string.
Note
Takes into account monospacing flag.
- Parameters
string – [in] Pointer to a string.
-
unsigned int gfx_GetCharWidth(const char c) __attribute__((__pure__))
Gets the pixel width of the given character.
Note
Takes into account monospacing flag.
- Parameters
c – [in] Character to get width of.
- Returns
Width in pixels of character.
-
void gfx_SetClipRegion(int xmin, int ymin, int xmax, int ymax)
Sets the dimensions of the drawing window for all clipped routines.
- Parameters
xmin – [in] Minimum x coordinate, inclusive (default 0).
ymin – [in] Minimum y coordinate, inclusive (default 0).
xmax – [in] Maximum x coordinate, exclusive (default 320).
ymax – [in] Maximum y coordinate, exclusive (default 240).
-
bool gfx_GetClipRegion(gfx_region_t *region)
Clips a region to fit within the drawing window using Cohen-Sutherland.
- Returns
False if offscreen, true if onscreen.
-
void gfx_ShiftDown(uint8_t pixels)
Shifts/Slides the drawing window down.
Note
Remnant data after a shift is undefined.
- Parameters
pixels – [in] Number of pixels to shift.
-
void gfx_ShiftUp(uint8_t pixels)
Shifts/Slides the drawing window up.
Note
Remnant data after a shift is undefined.
- Parameters
pixels – [in] Number of pixels to shift.
-
void gfx_ShiftLeft(uint24_t pixels)
Shifts/Slides the drawing window left.
Note
Remnant data after a shift is undefined.
- Parameters
pixels – [in] Number of pixels to shift.
-
void gfx_ShiftRight(uint24_t pixels)
Shifts/Slides the drawing window right.
Note
Remnant data after a shift is undefined.
- Parameters
pixels – [in] Number of pixels to shift.
-
uint16_t gfx_Lighten(uint16_t color, uint8_t amount) __attribute__((__const__))
Lightens a given 1555 color; useful for palette color conversions.
Note
0 returns full white, 255 returns original color.
- Parameters
color – [in] Original color input in 1555 format.
amount – [in] Amount to lighten by.
- Returns
Lightened color.
-
uint16_t gfx_Darken(uint16_t color, uint8_t amount) __attribute__((__const__))
Darkens a given 1555 color; useful for palette color conversions.
Note
0 returns full black, 255 returns original color.
- Parameters
color – [in] Original color input in 1555 format.
amount – [in] Amount to darken by.
- Returns
Darkened color.
-
void gfx_FloodFill(uint24_t x, uint8_t y, uint8_t color)
Fills an area with a color.
Note
This routine performs clipping to stay within the window, but you must ensure it starts in the window.
- Parameters
x – [in] X coordinate to begin filling at.
y – [in] Y coordinate to begin filling at.
color – [in] New color to fill with.
-
void gfx_RLETSprite(const gfx_rletsprite_t *sprite, int x, int y)
Draws a sprite with RLE transparency.
- Parameters
sprite – [in] sprite to draw.
x – [in] X coordinate.
y – [in] Y coordinate.
-
void gfx_RLETSprite_NoClip(const gfx_rletsprite_t *sprite, uint24_t x, uint8_t y)
Draws an unclipped sprite with RLE transparency.
- Parameters
sprite – [in] Sprite to draw.
x – [in] X coordinate.
y – [in] Y coordinate.
-
gfx_sprite_t *gfx_ConvertFromRLETSprite(const gfx_rletsprite_t *sprite_in, gfx_sprite_t *sprite_out)
Converts a sprite with RLE transparency to a sprite with normal transparency.
Width and height will be set in the converted sprite.
The transparent color index in the converted sprite is controlled by gfx_SetTransparentColor().
See also
See also
- Attention
The output sprite must have been allocated with a large enough
data
field to hold the converted sprite data, which will bewidth * height
bytes large.
- Parameters
sprite_in – [in] Input sprite with RLE transparency.
sprite_out – [out] Converted sprite with normal transparency.
- Returns
The converted sprite.
-
gfx_rletsprite_t *gfx_ConvertToRLETSprite(const gfx_sprite_t *sprite_in, gfx_rletsprite_t *sprite_out)
Converts a sprite with normal transparency to a sprite with RLE transparency.
Width and height will be set in the converted sprite.
The transparent color index in the input sprite is controlled by gfx_SetTransparentColor().
See also
- Attention
The output sprite must have been allocated with a large enough data field to hold the converted sprite data; see gfx_AllocRLETSprite() for information.
Note
To avoid needing to predict the output size and risking either the prediction being too high and wasting space, or being too low and corrupting memory, gfx_ConvertMallocRLETSprite() can be used instead to allocate the exact amount of necessary space for the converted sprite.
- Parameters
sprite_in – [in] Input sprite with normal transparency.
sprite_out – [out] Converted sprite with RLE transparency.
- Returns
The converted sprite.
-
gfx_rletsprite_t *gfx_ConvertToNewRLETSprite(const gfx_sprite_t *sprite_in, void *(*malloc_routine)(size_t)) __attribute__((__nonnull__(2)))
Converts a sprite with normal transparency to a sprite with RLE transparency, allocating the exact amount of necessary space for the converted sprite.
Allocates the memory with
malloc_routine
. Width and height will be set in the converted sprite. ReturnsNULL
upon allocation failure.The transparent color index in the input sprite is controlled by gfx_SetTransparentColor().
Remark
If using
malloc
as themalloc_routine
, gfx_ConvertMallocRLETSprite() can be used as a shortcut.Remark
A gfx_sprite_t can be converted into an appropriately large, already-allocated gfx_rletsprite_t using gfx_ConvertToRLETSprite().
See also
- Parameters
sprite_in – [in] Input sprite with normal transparency.
malloc_routine – [in] Malloc implementation to use.
- Returns
A newly allocated converted sprite with RLE transparency.
-
struct gfx_sprite_t
- #include <graphx.h>
Sprite (image) type.
Whether or not a sprite includes transparency is not explicitly encoded, and is determined only by usage. If used with transparency, transparent pixels are those with a certain color index, which can be set with gfx_SetTransparentColor().
Remark
Create at compile-time with a tool like convimg. Create at runtime (with uninitialized data) with gfx_MallocSprite(), gfx_UninitedSprite(), or gfx_TempSprite().
Note
Displaying a gfx_rletsprite_t (which includes transparency) is significantly faster than displaying a gfx_sprite_t with transparency, and should be preferred. However, gfx_rletsprite_t does not support transformations, such as flipping and rotation. Such transformations can be applied to a gfx_sprite_t, which can then be converted to a gfx_rletsprite_t for faster display using gfx_ConvertToNewRLETSprite() or gfx_ConvertToRLETSprite().
-
struct gfx_rletsprite_t
- #include <graphx.h>
Sprite (image) type with RLE transparency.
Remark
Create at compile-time with a tool like convimg.
- Attention
Displaying a gfx_rletsprite_t (which includes transparency) is significantly faster than displaying a gfx_sprite_t with transparency, and should be preferred. However, gfx_rletsprite_t does not support transformations, such as flipping and rotation. Such transformations can be applied to a gfx_sprite_t, which can then be converted to a gfx_rletsprite_t for faster display using gfx_ConvertToNewRLETSprite() or gfx_ConvertToRLETSprite().
-
struct gfx_point_t
- #include <graphx.h>
A structure for working with 2D points.
-
struct gfx_region_t
- #include <graphx.h>
Defines a rectangular graphics region.
See also
-
struct gfx_tilemap_t
- #include <graphx.h>
Defines tilemap structure.
See also
Public Members
-
uint8_t *map
Pointer to tilemap array.
-
gfx_sprite_t **tiles
Pointer to tileset sprites for the tilemap.
-
uint8_t tile_height
Individual tile height.
-
uint8_t tile_width
Individual tile width.
-
uint8_t draw_height
Number of tilemap rows to draw.
-
uint8_t draw_width
Number of tilemap columns to draw.
-
uint8_t type_width
Tile type height.
See also
-
uint8_t type_height
Tile type width.
See also
-
uint8_t height
Total number of rows in the tilemap.
-
uint8_t width
Total number of columns in the tilemap.
-
uint8_t y_loc
Y pixel location on the screen for the tilemap.
-
uint24_t x_loc
X pixel location on the screen for the tilemap.
-
uint8_t *map